Understanding the Differences Between T-SQL, SQL Server and SQL
Table of Contents
Introduction:
Differences Between T-SQL and SQL When working with databases, terms like SQL, T-SQL, and SQL Server often come up. While they are related, they refer to different aspects of database management and querying. Understanding these differences is crucial for database professionals, developers, and anyone involved in data management. Below is a detailed explanation of each term and how they differ.
1. SQL (Structured Query Language)
SQL, or Structured Query Language, is the standard Differences Between T-SQL and SQL language used to communicate with relational databases. Differences Between T-SQL and SQL It is a domain-specific language designed for managing and manipulating data held in a relational database management system (RDBMS). Differences Between T-SQL and SQL SQL is used to perform tasks such as:
- Querying Data: Retrieving data from a database using SELECT statements.
- Inserting Data: Adding new records to a database using Differences Between T-SQL and SQL INSERT statements.
- Updating Data: Modifying existing data in a database with UPDATE statements.
- Deleting Data: Removing records from a database using DELETE Differences Between T-SQL and SQL statements.
- Creating and Managing Database Objects: Using CREATE, ALTER, and DROP statements to manage tables, views, indexes, Differences Between T-SQL and SQL and other database objects.
SQL is a standard adopted by many database systems, such as MySQL, PostgreSQL, Oracle, and Microsoft SQL Server. However, while the core syntax and functionality are Differences Between T-SQL and SQL consistent, each database system may implement extensions or variations of SQL.
What is SQL (Structured Query Language)?
SQL (Structured Query Language) is a standardized programming language specifically designed Differences Between T-SQL and SQL for managing and manipulating relational databases. It serves as the primary tool for interacting with databases, allowing users to perform a wide range of data-related tasks. SQL is used across various relational database management systems (RDBMS) like MySQL, PostgreSQL, Oracle, Microsoft SQL Server, and others.
Key Functions of SQL
- Querying Data:
- SELECT Statement: SQL is most commonly used to retrieve data from a database. The SELECT statement is used to specify which data you want to fetch from one or more tables. It allows for filtering, sorting, and grouping of data.
Example:
sql
Copy code
SELECT first_name, last_name FROM employees WHERE department = ‘Sales’;
- This query retrieves the first and last names of employees working in the Sales department.
- Inserting Data:
- INSERT Statement: SQL allows you to add new records to a table using the INSERT INTO statement.
Example:
sql
Copy code
INSERT INTO employees (first_name, last_name, department) VALUES (‘John’, ‘Doe’, ‘Marketing’);
- This query adds a new employee named John Doe to the Marketing department.
- Updating Data:
- UPDATE Statement: You can modify existing records in a database with the UPDATE statement.
Example:
sql
Copy code
UPDATE employees SET department = ‘Sales’ WHERE employee_id = 1001;
- This query updates the department of the employee with ID 1001 to Sales.
- Deleting Data:
- DELETE Statement: SQL allows you to remove records from a table using the DELETE statement.
Example:
sql
Copy code
DELETE FROM employees WHERE employee_id = 1001;
- This query deletes the employee with ID 1001 from the employees’ table.
- Creating and Managing Database Objects:
- CREATE Statement: SQL is also used to define and manage the structure of the database, including tables, indexes, views, and more.
Example:
sql
Copy code
CREATE TABLE departments (
department_id INT PRIMARY KEY,
department_name VARCHAR(50)
);
- This query creates a new table named departments with two columns: department_id and department_name.
- Controlling Access to Data:
- GRANT and REVOKE Statements: SQL includes commands to control who can access and modify data in the database. The GRANT and REVOKE statements are used to assign and remove permissions.
Example:
sql
Copy code
GRANT SELECT ON employees TO user_name;
- This query gives a user permission to read data from the employees table.
SQL: A Standard Language
SQL is governed by international standards, such as those set by the American National Standards Institute (ANSI) and the International Organization for Standardization (ISO). These standards ensure that the basic syntax and functionality of SQL remain consistent across different database systems, although each system may implement extensions or variations of SQL.
Common SQL Clauses and Commands
- WHERE: Used to filter records based on specified conditions.
- ORDER BY: Used to sort the result set by one or more columns.
- GROUP BY: Used to group rows that have the same values in specified columns.
- HAVING: Used to filter groups based on a condition.
- JOIN: Used to combine rows from two or more tables based on a related column.
Importance of SQL
SQL is the backbone of data management in relational databases. Its ability to handle large volumes of data efficiently, combined with its powerful querying capabilities, makes it indispensable for tasks such as:
- Data Retrieval: Extracting meaningful information from databases to support decision-making.
- Data Analysis: Performing aggregations, calculations, and data transformations.
- Data Maintenance: Keeping the data up-to-date and accurate.
- Database Administration: Defining the structure of databases and managing permissions.
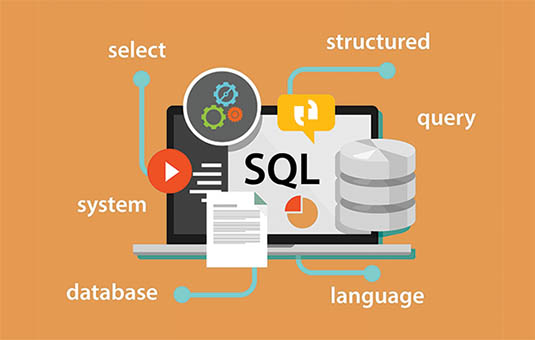
2. T-SQL (Transact-SQL)
T-SQL, or Transact-SQL, is an extension of SQL developed by Microsoft. It is the proprietary Differences Between T-SQL and SQL procedural language used in Differences Between T-SQL and SQL Microsoft SQL Server, adding more capabilities to the standard SQL language. These enhancements make T-SQL more powerful, allowing for:
- Procedural Programming: T-SQL supports procedural programming constructs such as variables, loops, and conditional statements (IF, WHILE, BEGIN…END blocks), which are not part of standard SQL.
- Error Handling: T-SQL provides mechanisms for handling errors with TRY…CATCH blocks, which help in building Differences Between T-SQL and SQL Differences Between T-SQL and SQL robust database applications.
- Advanced Functions: T-SQL includes additional built-in functions, such as system functions (GETDATE(), DATEPART(), etc.) and string manipulation functions (CHARINDEX(), PATINDEX()).
- Extended Capabilities: T-SQL extends SQL with Differences Between T-SQL and SQL additional features like Common Table Expressions (CTEs), dynamic SQL, and more advanced querying capabilities.
T-SQL is essential for developers Differences Between T-SQL and SQL working with SQL Server, as it allows for the creation of more complex and Differences Between T-SQL and SQL powerful queries and stored procedures.
What is T-SQL (Transact-SQL)?
T-SQL (Transact-SQL) is an extension of the SQL (Structured Query Language) developed by Microsoft, specifically for use with Microsoft SQL Server. While SQL is the standard language for querying and managing relational databases, T-SQL adds additional features and capabilities, making it more powerful for complex database operations, procedural programming, and advanced data manipulation.
Key Features of T-SQL
- Procedural Programming Constructs:
- Unlike standard SQL, T-SQL includes procedural programming constructs that allow for more complex logic within queries and scripts. This includes:
- Variables: Store data values that can be reused within a script.
- Control-of-Flow Statements: Conditional statements (IF…ELSE) and loops (WHILE, BEGIN…END) enable the execution of code based on specific conditions.
- Unlike standard SQL, T-SQL includes procedural programming constructs that allow for more complex logic within queries and scripts. This includes:
Example:
sql
Copy code
DECLARE @TotalSales INT;
SET @TotalSales = (SELECT SUM(sales_amount) FROM sales WHERE sales_date = ‘2024-08-01’);
IF @TotalSales > 10000
BEGIN
PRINT ‘Sales are above target!’;
END
ELSE
BEGIN
PRINT ‘Sales are below target.’;
END
- Error Handling:
- T-SQL provides robust error handling capabilities with TRY…CATCH blocks. This allows developers to handle exceptions and errors gracefully, ensuring that database operations do not fail silently.
Example:
sql
Copy code
BEGIN TRY
— Attempt to execute this block
UPDATE accounts SET balance = balance – 100 WHERE account_id = 123;
END TRY
BEGIN CATCH
— Handle the error here
PRINT ‘An error occurred during the transaction.’;
END CATCH
- Built-in Functions and Operators:
- T-SQL includes a wide array of built-in functions that extend the capabilities of standard SQL. These include:
- String Functions: Functions like LEN(), CHARINDEX(), and PATINDEX() for string manipulation.
- Date and Time Functions: Functions like GETDATE(), DATEPART(), and DATEDIFF() to work with date and time values.
- Mathematical Functions: Functions like ROUND(), CEILING(), and FLOOR() for mathematical operations.
- T-SQL includes a wide array of built-in functions that extend the capabilities of standard SQL. These include:
Example:
sql
Copy code
SELECT UPPER(first_name), DATEDIFF(YEAR, birth_date, GETDATE()) AS age
FROM employees
WHERE department = ‘HR’;
- This query converts the first name to uppercase and calculates the age of employees in the HR department.
- Advanced Querying Capabilities:
- T-SQL enhances SQL with additional querying features such as:
- Common Table Expressions (CTEs): Temporary result sets that can be referenced within a SELECT, INSERT, UPDATE, or DELETE statement.
- Window Functions: Functions like ROW_NUMBER(), RANK(), and OVER() that perform calculations across a set of table rows related to the current row.
- T-SQL enhances SQL with additional querying features such as:
Example:
sql
Copy code
WITH SalesRanking AS (
SELECT employee_id, sales_amount,
RANK() OVER (ORDER BY sales_amount DESC) AS SalesRank
FROM sales
)
SELECT * FROM SalesRanking WHERE SalesRank <= 5;
- This query ranks employees by their sales amount and retrieves the top 5.
- Stored Procedures and Triggers:
- Stored Procedures: T-SQL allows you to write stored procedures, which are precompiled collections of T-SQL code that can be executed as a single unit. This is useful for encapsulating business logic and reusing code.
- Triggers: T-SQL supports triggers, which are special types of stored procedures that automatically execute in response to certain events on a table, such as INSERT, UPDATE, or DELETE operations.
Example of Stored Procedure:
sql
Copy code
CREATE PROCEDURE UpdateEmployeeSalary
@EmployeeID INT,
@NewSalary DECIMAL(10, 2)
AS
BEGIN
UPDATE employees SET salary = @NewSalary WHERE employee_id = @EmployeeID;
END;
- This stored procedure updates the salary of a specific employee.
- Dynamic SQL:
- T-SQL supports dynamic SQL, which allows you to construct and execute SQL statements dynamically at runtime. This is particularly useful when you need to execute varying SQL commands based on user input or other conditions.
Example:
sql
Copy code
DECLARE @SQL NVARCHAR(1000);
SET @SQL = ‘SELECT * FROM ‘ + @TableName;
EXEC sp_executesql @SQL;
- This script dynamically constructs and executes a SELECT statement on a table specified by the variable @TableName.
Use Cases for T-SQL
- Complex Data Manipulation: T-SQL’s procedural features are ideal for tasks that require complex logic, such as batch processing, data transformation, and conditional data updates.
- Automating Database Tasks: T-SQL is commonly used to automate routine database tasks, including backups, maintenance, and data imports.
- Building Applications: Developers use T-SQL to create stored procedures, triggers, and views that encapsulate business logic, making application development more efficient and secure.
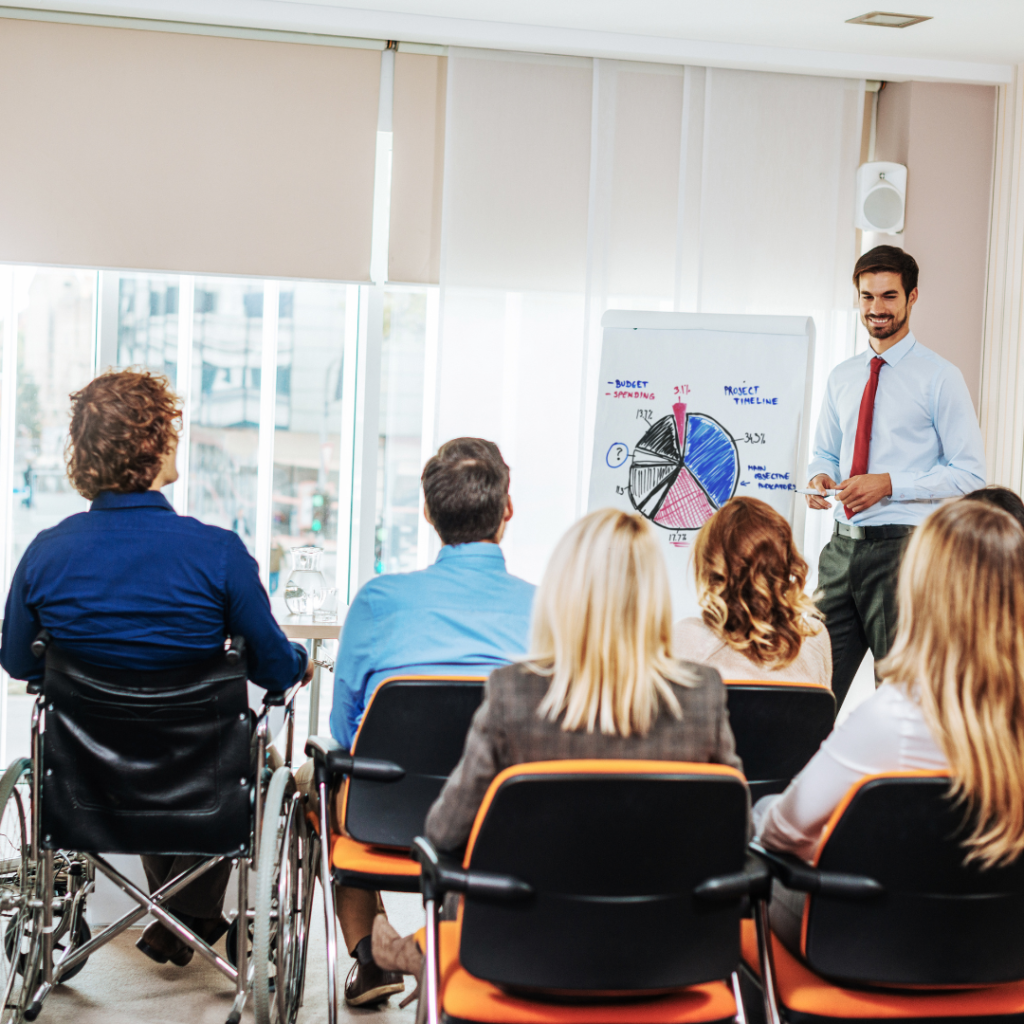
3. SQL Server
SQL Server is a relational database management system (RDBMS) developed by Microsoft. It is a full-featured database platform that uses T-SQL as its primary query language. SQL Server provides a comprehensive environment for data storage, processing, and security, offering features such as:
- Data Storage and Management: SQL Server efficiently stores and manages large volumes of data, supporting tables, indexes, views, and more.
- Security Features: It offers robust security features, including encryption, role-based access control, and auditing.
- Performance Optimization: SQL Server includes tools and features for performance tuning, indexing, and query optimization.
- High Availability and Disaster Recovery: SQL Server supports high availability features like Always On Availability Groups, database mirroring, and replication.
- Integration Services: SQL Server integrates with various Microsoft services, such as Azure, Active Directory, and Power BI, enabling seamless data connectivity and analytics.
SQL Server is designed for enterprise-level applications, providing a scalable and secure platform for managing databases.
What is SQL Server?
SQL Server is a relational database management system Differences Between T-SQL and SQL (RDBMS) developed by Microsoft. It is designed to store, retrieve, and manage data as requested by other software applications, whether they are running on the same computer or across a network. SQL Server is widely used in enterprise environments for a variety of data-intensive applications, including business intelligence, data warehousing, and online transaction processing (OLTP).
Key Features of SQL Server
- Relational Database Management:
- SQL Server is a full-featured RDBMS that supports the creation, maintenance, and use of relational databases. It allows you to store data in structured tables, define relationships Differences Between T-SQL and SQL between tables, and enforce data integrity through constraints, keys, and indexes.
- Example: You can create tables, define relationships, and enforce rules such as foreign key constraints to ensure referential integrity between related tables.
- T-SQL (Transact-SQL):
- SQL Server uses T-SQL (Transact-SQL) as its primary query language. T-SQL extends standard SQL with additional features for procedural programming, error handling, and advanced querying capabilities.
- Example: Using T-SQL, you can write complex queries, stored procedures, and triggers that automate and enforce business logic within the database.
- Data Security and Compliance:
- SQL Server provides robust security features to protect data, including encryption, authentication, authorization, and auditing. It supports role-based access control (RBAC), transparent data encryption (TDE), and Always Encrypted technology, which ensures sensitive data is protected both at rest and in transit.
- Example: You can encrypt sensitive data in columns using Differences Between T-SQL and SQL Always Encrypted, ensuring that even database administrators cannot view the data without the necessary keys.
- High Availability and Disaster Recovery:
- SQL Server includes features to ensure high availability and disaster recovery (HA/DR). These features help minimize downtime and data loss in case of hardware failure, natural disasters, or other catastrophic events. Key HA/DR features include:
- Always On Availability Groups: Provides high availability and disaster recovery for a set of databases.
- Failover Clustering: Ensures that SQL Server instances remain available in case of server failure.
- Log Shipping and Database Mirroring: Techniques for replicating and synchronizing data across servers to ensure data continuity.
- Example: You can configure an Always On Availability Group to replicate your database across multiple servers, ensuring that your data remains available even if one server goes down.
- SQL Server includes features to ensure high availability and disaster recovery (HA/DR). These features help minimize downtime and data loss in case of hardware failure, natural disasters, or other catastrophic events. Key HA/DR features include:
- Performance and Scalability:
- SQL Server is built to handle large volumes of data and high transaction rates, making it suitable for enterprise-level Differences Between T-SQL and SQL applications. It includes features such as:
- In-Memory OLTP: Accelerates transaction processing by storing critical tables in memory.
- Columnstore Indexes: Improve query performance for large datasets by storing data in a compressed columnar format.
- Query Store: Captures a history of query executions and performance metrics, helping to identify and address performance Differences Between T-SQL and SQL issues.
- Example: You can use In-Memory OLTP to speed up transactions in a high-volume system, reducing latency and improving performance.
- SQL Server is built to handle large volumes of data and high transaction rates, making it suitable for enterprise-level Differences Between T-SQL and SQL applications. It includes features such as:
- Integration Services:
- SQL Server provides tools for integrating, transforming, and migrating data between different systems. SQL Server Integration Services (SSIS) is a platform for building data integration and workflow applications. SSIS can be used for tasks such as data migration, data warehousing, and ETL (Extract, Transform, Load) processes.
- Example: You can use SSIS to Differences Between T-SQL and SQL extract data from multiple sources, transform it according to business rules, and load it into a data warehouse for analysis.
- Business Intelligence (BI) Tools:
- SQL Server includes tools for business intelligence and data analysis, such as SQL Server Reporting Services (SSRS) and SQL Server Analysis Services (SSAS). These tools allow organizations to generate reports, perform data analysis, and create multidimensional databases (cubes) for advanced analytics.
- Example: Using SSAS, you can create a cube that allows users to perform fast, interactive analysis of large datasets, with the ability to drill down into detailed data.
- Integration with Microsoft Ecosystem:
- SQL Server seamlessly integrates with other Microsoft products, such as Azure cloud services, Differences Between T-SQL and SQL Power BI for data visualization, and Active Directory for user management and security. This integration makes SQL Server a key component in Microsoft’s broader data platform and cloud strategy.
Example: You can use Power BI to create interactive dashboards and reports that connect directly to SQL Server, providing real-time insights into your data.
- Relational Database Management:
Editions of SQL Server
SQL Server is available in several Differences Between T-SQL and SQL editions, each designed to meet different needs:
- Enterprise Edition: The most comprehensive edition, offering full functionality for large enterprises, including advanced HA/DR, BI, and analytics Differences Between T-SQL and SQL features.
- Standard Edition: A more cost-effective option for medium-sized organizations, with many of the core features of the Enterprise Edition, but with some limitations on scalability and performance.
- Express Edition: A free, entry-level editionDifferences Between T-SQL and SQL with basic features, suitable for small-scale applications and development purposes.
- Developer Edition: Provides all the features of the Enterprise Edition but is licensed only for development and testing, not for production use.
Use Cases for SQL Server
- Enterprise Applications: SQL Server is commonly used to power enterprise applications that require reliable, high-performance data storage and management.
- Data Warehousing and Business Intelligence: Organizations use SQL Server for building data warehouses and performing BI tasks, allowing them to analyze large volumes of data and make informed decisions.
- Web Applications: SQL Server is often used as the backend database for web applications, providing a scalable and secure platform for managing user data, transactions, and content.
SQL Server is a relational database management system (RDBMS) developed by Microsoft. It is a comprehensive platform for data storage, retrieval, and management, designed to support a wide range of applications, from small desktop systems to large-scale enterprise applications. SQL Server is more than just a database engine; it includes various tools and services for data management, business intelligence, and analytics.
Key Components and Features of SQL Server:
- Database Engine: Differences Between T-SQL and SQL The core component of SQL Server, responsible for data storage, processing, and security. It manages the physical storage of data, executes queries, and enforces data integrity.
- Example: SQL Server can store and manage data across multiple tables with complex relationships, ensuring that the data remains consistent and secure.
- Integration with T-SQL: SQL Server uses T-SQL as its primary query language, enabling users to write complex queries, stored procedures, and triggers directly within the database environment.
- Security Features: SQL Server offers robust security features, including encryption, authentication, and authorization, to protect data. It supports role-based access control (RBAC) and integrates with Active Directory for user management.
- Example: You can enforce data encryption at the column level using Always Encrypted, ensuring sensitive data remains protected even if the database is compromised.
- High Availability and Disaster Recovery: SQL Server includes features like Always On Availability Groups, failover clustering, and log shipping to ensure data availability and minimize downtime.
- Example: Always On Availability Groups allow you to replicate databases across multiple servers, ensuring that data remains accessible even if one server fails.
- Business Intelligence Tools: SQL Server includes SQL Server Reporting Services (SSRS) for report generation, SQL Server Integration Services (SSIS) for data integration, and SQL Server Analysis Services (SSAS) for multidimensional analysis and data mining.
- Example: SSIS can be used to extract data from various sources, transform it according to business rules, and load it into a data warehouse for analysis.
- Performance and Scalability: SQL Server is designed to handle large volumes of data and high transaction rates, making it suitable for enterprise-level applications. Features like In-Memory OLTP and Columnstore indexes help optimize performance.
- Example: In-Memory OLTP can be used to speed up transaction processing by storing frequently accessed data in memory.
- Editions: SQL Server comes in various editions, such as Enterprise, Standard, Express, and Developer, each catering to different use cases and budgets.
- Example: The Enterprise Edition offers full-featured capabilities for large organizations, while the Express Edition is free and suitable for small applications or development purposes.
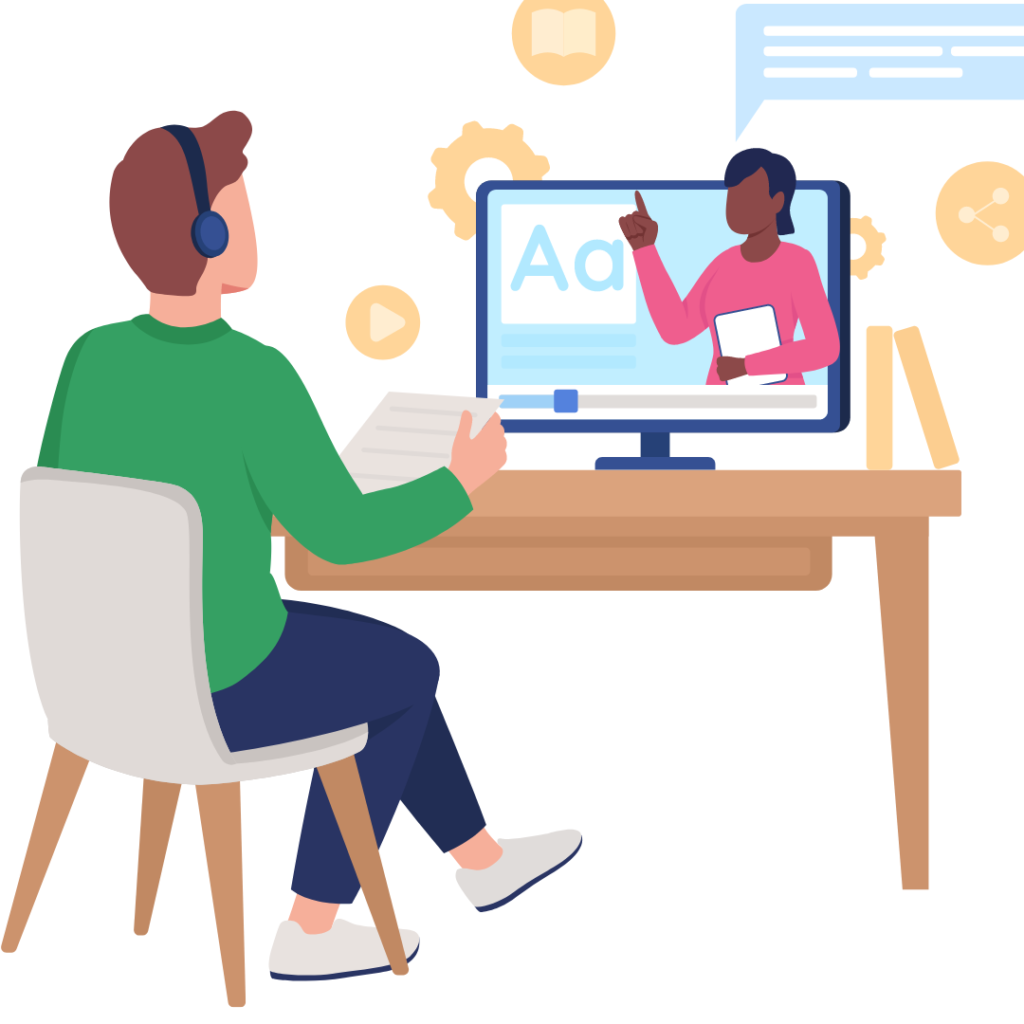
Conclusion:
SQL is an essential tool for anyone working Differences Between T-SQL and SQL with relational databases, Differences Between T-SQL and SQL from data analysts to database administrators and developers. Its simplicity, combined with its powerful capabilities, makes it accessible Differences Between T-SQL and SQL yet robust enough to handle complex data operations.
T-SQL is a powerful extension Differences Between T-SQL and SQL of SQL, adding procedural programming features, error handling, and advanced querying capabilities. It is tightly integrated with Microsoft SQL Server, making it a key tool for anyone developing Differences Between T-SQL and SQL or managing databases on this platform. T-SQL’s ability to handle complex logic, automate tasks, and enhance SQL’s functionality makes it indispensable for database professionals working within the Microsoft ecosystem.
SQL Server is a robust and versatile RDBMS that plays a critical role Differences Between T-SQL and SQL in many organizations’ data management strategies. With its comprehensive feature set, including advanced security, high availability, performance optimization, and integration with Microsoft’s broader ecosystem, SQL Server is well-suited for a wide range of applications, from small business solutions to Differences Between T-SQL and SQL large-scale enterprise systems.
SQL Server FAQs:
- What is SQL Server, and what is it used for?
- SQL Server Differences Between T-SQL and SQL is a relational database management system (RDBMS) developed by Microsoft. It is used to store, manage, and retrieve data for various applications, ranging from small-scale solutions to large enterprise-level systems.
- What are the different editions of SQL Server?
- SQL Server is available Differences Between T-SQL and SQL in several editions, including Enterprise, Standard, Express, and Developer editions. Each edition offers different features and capabilities, tailored to meet the needs of various users and organizations.
- How can I back up my SQL Server database?
- SQL Server allows you to back up your database using SQL Server Management Studio (SSMS) or T-SQL commands. You can perform full, differential, and transaction log backups to ensure data recovery in case of a failure.
- What are Always On Availability Groups in SQL Server?
- Always On Availability Groups is a high-availability and disaster recovery feature in SQL Server that provides failover capabilities for a group of databases. It allows you to ensure data availability and redundancy across multiple servers.
- How does SQL Server integrate with Azure?
- SQL Server can be integrated with Microsoft Azure to enable cloud-based solutions, such as Azure SQL Database, Azure SQL Managed Instance, and hybrid cloud environments. This Differences Between T-SQL and SQL integration allows for scalability, disaster recovery, and cost-effectiveness.
T-SQL FAQs:
- What is T-SQL, and how does it differ from SQL?
- T-SQL (Transact-SQL) is an extension of SQL used by Microsoft SQL Server. It includes additional features like procedural programming, error Differences Between T-SQL and SQL handling, and advanced querying capabilities that go beyond standard SQL.
- How do I create a stored procedure in T-SQL?
- A stored procedure in T-SQL is created using the CREATE PROCEDURE statement, allowing you to encapsulate and reuse complex business logic within the database. Stored procedures Differences Between T-SQL and SQL can include T-SQL statements, variables, and control-of-flow elements.
- What is the purpose of the TRY…CATCH block in T-SQL?
- The TRY…CATCH block in T-SQL is used for error handling. It allows you to catch and handle exceptions that occur during the execution of T-SQL code, ensuring that your application can gracefully manage errors.
- How can I improve the performance of my T-SQL queries?
- Improving T-SQL query performance can involve several techniques, such as optimizing indexes, avoiding unnecessary columns in SELECT statements, using appropriate joins, and Differences Between T-SQL and SQL analyzing execution plans to identify bottlenecks.
- What are Common Table Expressions (CTEs) in T-SQL?
- Common Table Expressions (CTEs) are temporary result sets that can be referenced within a SELECT, INSERT, UPDATE, or DELETE statement. CTEs are useful for simplifying complex queries and improving readability.